Basic Data Structures for a beginner web developer
Every programming newbie hears from more experienced developers that the fundamentals of coding are:
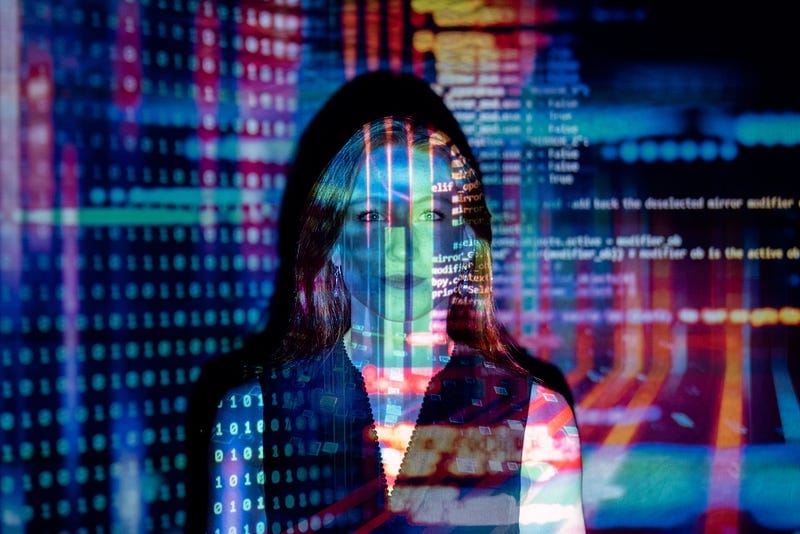
Every programming newbie hears from more experienced developers that the fundamentals of coding are:
- Data Structures,
- Algorithms
There are even unlimited memes in the Internet about that, how this basics are very unattractive. Every newbie dreams about creating one million dollar solution or an app, but…Firstly, let’s dive into “Why fundamentals are so important”
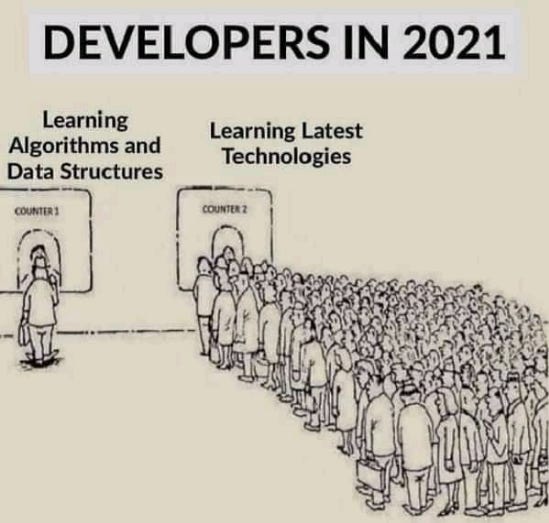
Data structures are not difficult
It sounds ridiculous, but basic knowledge of data structures is pretty easy. It does not require practicing hundreds of hours to create amazing apps. From my perspective, when I was starting coding in the university, by learning C and C++, it was a horror. I hated opening an IDE and implementing some new solutions, which were totally without bigger sense.
After graduating I met web developing, this tech branch really opened my eyes. I saw that coding is not super complicated, with basic free tutorial it is easy to code first app, even complicated like social media platform. However, thanks to the boring lectures in the university, I knew basics and it was easier to boost my dev career. By the basics, I mean Data structures.
What are data structures
Data is practically, the most powerful tool for businesses, organizations or even countries. Without efficient collecting data and operating on them, there is no programming.
Imagine creating a program which store no data, generally impossible. That is one of the most reasons for mastering basics of data structures.
Easily said, the data structure is a collection of data (values), the relations among them and operations (functions) that can be applied to the data.
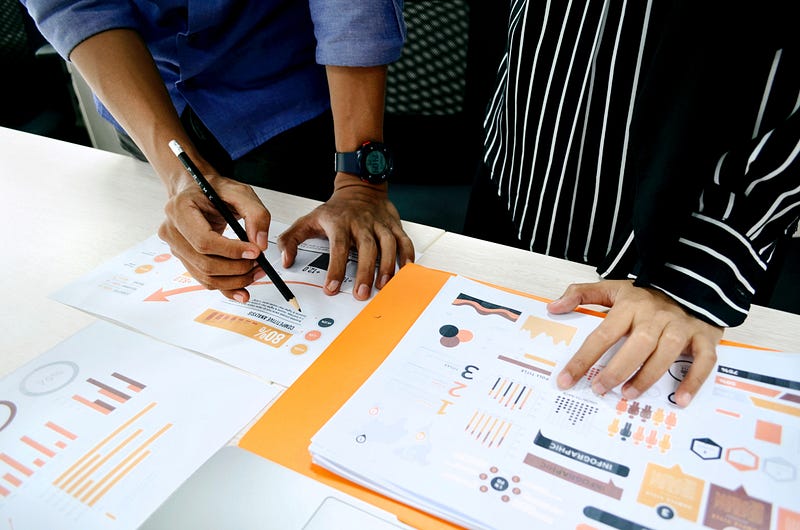
Basic Examples
To start creating useful apps, generally you need to know examples presented below:
- Arrays
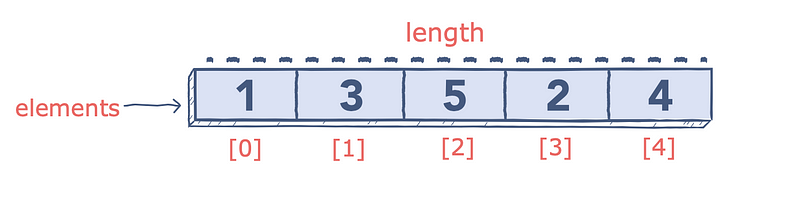
In simple words, array is a special variable, which can store more than one value:const apps = ["Instagram", "Facebook", "Twitter"];
It would be extremely unefficient to store multiple items in different variables, like presented below:let app1 = "Instagram";
let app2 = "Facebook";
let app3 = "Twitter";
Imagine using hundreds of variables, it would be just impossible and very annoying.
When I want to access the specific value, it is possible by using syntax presented below:let myfavouriteApp = apps[0];console.log(myFavouriteApp);
// there will be printed - "Instagram"
Remember that in arrays, the very first element has an index 0.
- Objects

Objects are very important in web developing, it is practically impossible to make a clean and useful platform without them. The easiest explanation of an object is like that:
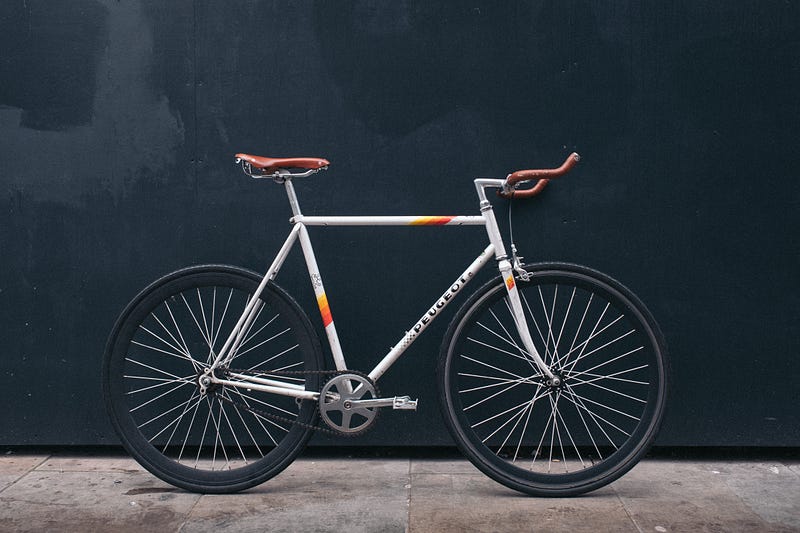
In real life, the bike can be described as an object.
Bike can have properties like: weight, color, model and methods like start, stop.
All bikes have the same properties, but the values are different, depending on a concrete model and type.const bike = {type:"Peugeot", model:"500", color:"silver", weight: 10};
Example above presents how to define a bike object in javaScript.
Accessing a concrete property of an object is very easy and intuitive, if there is a need for printing, for example a type of a bike object, it is done in the way showed below.const typeOfBike = bike.type;console.log(typeOfBike);
// there will be printed - "Peugeot"
- Queues
Simply, a queue is a list, but it process elements in the order of they were entered. Queues can be thought as a FIFO (First In, First Out) version of Stacks.
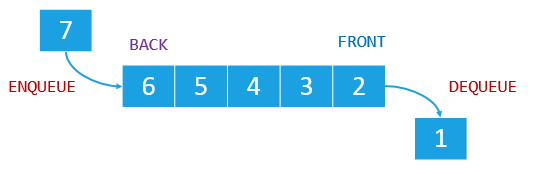
To have a great visualization, let’s consider queues like a single tunnel with only one way: the first car to enter is the first car to exit. There is no other way.
- Linked List
Linked lists does not use physical placement of data in memory. In arrays there are used indexes or positions. Linked lists are basing on referencing system, elements are stored in different nodes which indicates to the next node, that is how it is linked.
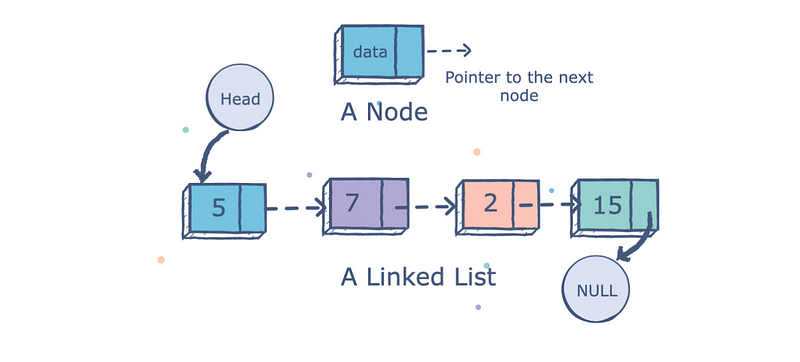
- Trees
Trees and graphs are less common in development, especially for beginners. However It is worth to mention that those data structures are available to use.
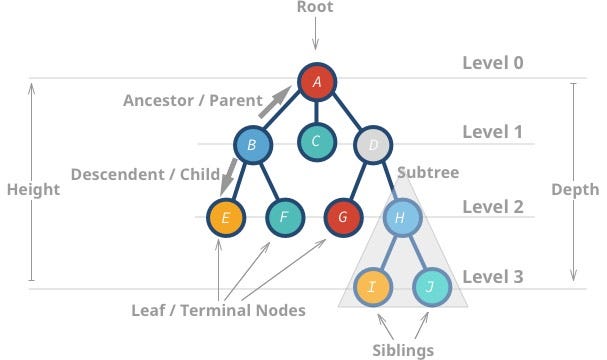
Easily said, trees are also relation-based, but represent hierarchical structures. In linked lists it could be caught, that it was basing on nodes. Trees have the root node from which are “connected” branch nodes. They are also called parent nodes, if next child nodes are attached to them.
Trees can have multiple levels, it can be determined as a depth. If tree is very complex, there can be added subtrees.
Entire structure is shown in an image above.
- Graphs
Graphs are relation-based data structure which are extremely helpful for storing web-like relationships.
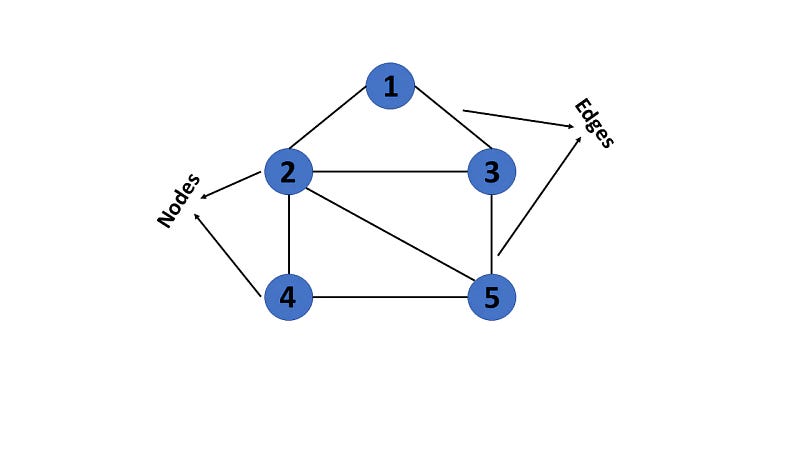
A graph is a a non-linear, made up of nodes and edges kind of data structure. Graphs can be: infinite, trivial, simple, multi, and many more.
- Hash Tables
Hash tables are a kind of data structure that allow creating a list of paired values. Retrieving a concrete value is possible by using the key for that value.
Hash tables transform key into an integer index using hash functions and the index will indicate where to store the pair key/value in a memory.
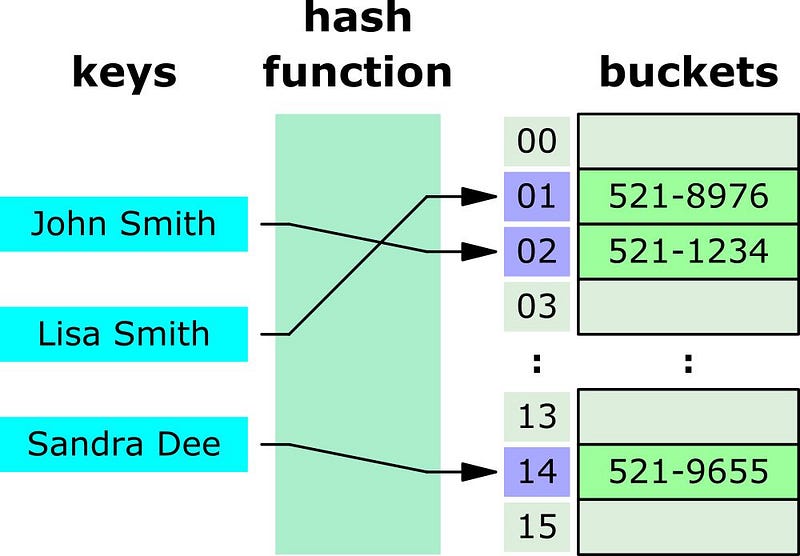
Conclusion
Knowledge of data structures is crucial in web development, especially arrays and objects. Without those two it is impossible to build basic, functional apps. Getting acquainted with all the rest positions in this article will help in improving even simple, but complex platforms. Sometimes it can be more efficient to use linked lists, trees, graphs instead of arrays and objects.
Hash tables are super important, even crucial to know, before learning blockchain, which is super perspective in next years of development.
For all newbies, It is highly recommended to learn and use in different conditions arrays and objects, to master it in the very high level and after developing complex apps, try to implement more advanced structures.